Useful Patterns and Tricks in Golang Development
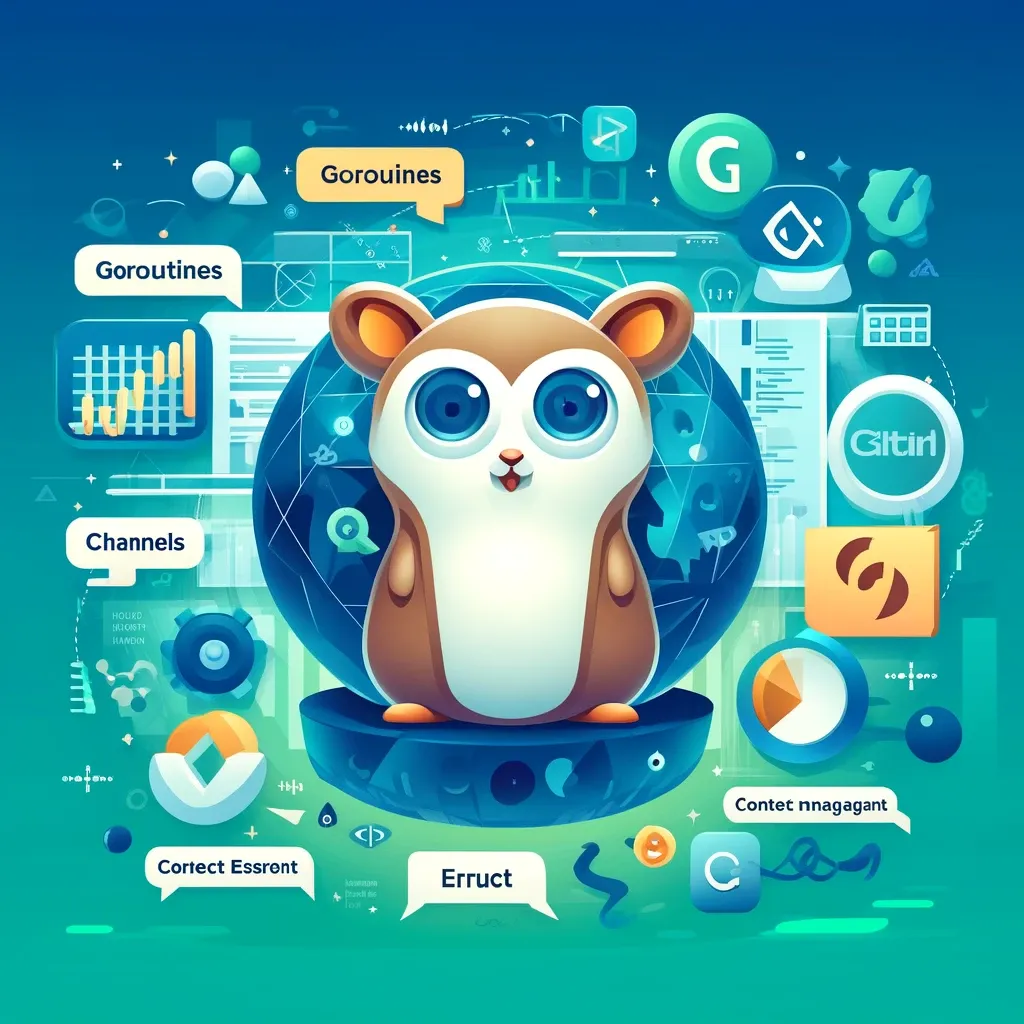
Golang, or Go, is a statically typed, compiled language designed by Google. It has gained popularity for its simplicity, efficiency, and powerful concurrency features. As you dive deeper into Go development, you'll discover several patterns and tricks that can significantly enhance your productivity and code quality. In this post, we'll explore some of these useful patterns and tricks.
1. Effective Use of Goroutines and Channels
Goroutines
Goroutines are lightweight threads managed by the Go runtime. They are a fundamental feature for achieving concurrency in Go. You can start a goroutine by simply prefixing a function or method call with the go
keyword.
go func()
{ // Your concurrent code here
}()
Channels
Channels provide a way for goroutines to communicate with each other and synchronize their execution. Use channels to send and receive messages between goroutines.
ch := make(chan int
)go func()
{ ch <- 42
}()
value := <-chfmt.Println(value) // Output: 42
2. The select
Statement
The select
statement in Go allows a goroutine to wait on multiple communication operations. It’s like a switch statement for channels.
select
{case
msg1 := <-ch1: fmt.Println("Received"
, msg1)case
msg2 := <-ch2: fmt.Println("Received"
, msg2)case <-time.After(time.Second * 5
): fmt.Println("Timeout"
)
}
3. Struct Embedding
Struct embedding is a powerful feature that allows you to create complex types by combining simpler ones. It promotes code reuse and can help you compose functionalities cleanly.
type Animal struct
{ Name string
}func (a *Animal)
Speak() { fmt.Println("Animal speaks"
)
}type Dog struct
{
Animal
}func main()
{
d := Dog{} d.Name = "Fido"
d.Speak() // Output: Animal speaks
}
4. Error Handling Patterns
Custom Error Types
Creating custom error types can provide more context about the error, making it easier to handle different error scenarios.
type MyError struct
{ ErrCode int
ErrMsg string
}func (e *MyError) Error() string
{ return fmt.Sprintf("Error %d: %s"
, e.ErrCode, e.ErrMsg)
}func someFunction() error
{ return &MyError{ErrCode: 123, ErrMsg: "Something went wrong"
}
}
Wrapping Errors
The fmt.Errorf
function can be used to wrap errors with additional context.
func someFunction() error
{
err := anotherFunction() if err != nil
{ return fmt.Errorf("someFunction: %w"
, err)
} return nil
}
5. Context Package
The context
package is essential for managing deadlines, cancellations, and other request-scoped values across API boundaries and goroutines.
ctx, cancel := context.WithTimeout(context.Background(), time.Second*10
)defer
cancel()select
{case <-time.After(time.Second * 11
): fmt.Println("operation timed out"
)case
<-ctx.Done(): fmt.Println("context canceled"
)
}
6. Testing and Benchmarking
Golang provides built-in support for testing and benchmarking through the testing
package.
Writing Tests
import "testing"
{
func TestAdd(t *testing.T) result := Add(2, 3
) if result != 5
{ t.Errorf("expected 5, got %d"
, result)
}
}
Writing Benchmarks
func BenchmarkAdd(b *testing.B)
{ for i := 0
; i < b.N; i++ { Add(2, 3
)
}
}
7. Using defer
for Cleanup
The defer
statement ensures that a function call is performed later in a program’s execution, usually for purposes of cleanup.
func readFile(filename string) (string, error
) {
file, err := os.Open(filename) if err != nil
{ return ""
, err
} defer
file.Close() // Read the file
}
8. Interfaces for Dependency Injection
Interfaces in Go allow you to define methods that your types must implement, making your code more flexible and easier to test.
type Notifier interface
{ Notify(message string) error
}type EmailNotifier struct
{}func (e *EmailNotifier) Notify(message string) error
{ // Send email
return nil
}func SendNotification(n Notifier, message string) error
{ return
n.Notify(message)
}
Conclusion
These patterns and tricks can significantly enhance your Golang development experience, making your code more efficient, maintainable, and easier to read. As you continue to work with Go, you'll find even more idiomatic ways to solve problems and improve your workflow. Happy coding!