Effective Use Cases for Goroutines and Channels in Golang
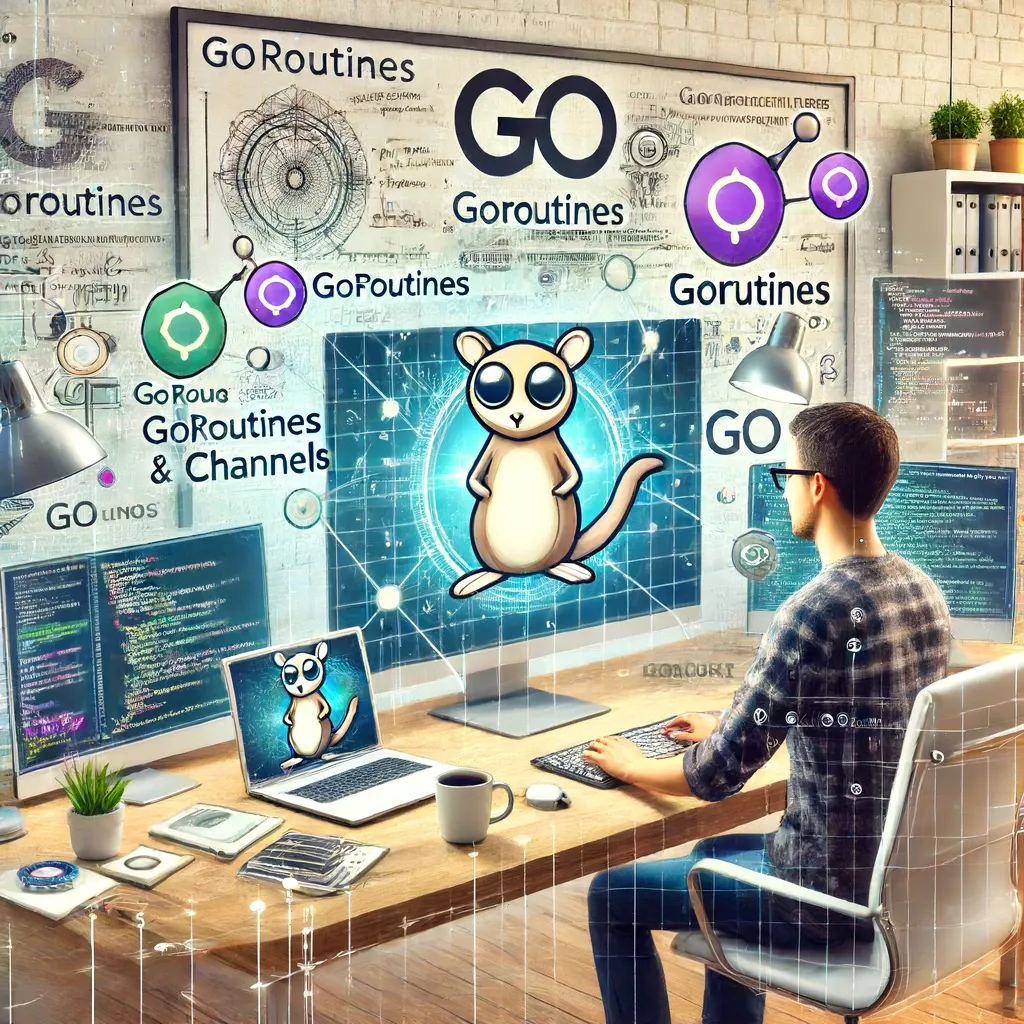
Golang, often referred to as Go, is renowned for its simplicity and efficiency, especially in handling concurrent tasks. One of its most powerful features is the use of goroutines and channels for concurrent programming. In this blog post, we'll explore some practical use cases for goroutines and channels to help you harness their full potential.
1. Asynchronous Task Execution
Goroutines are lightweight threads managed by the Go runtime, making them ideal for running tasks asynchronously. This allows the main program to continue executing without waiting for the task to complete.
package
mainimport
( "fmt"
"time"
)func printNumbers()
{ for i := 1; i <= 5
; i++ {
fmt.Println(i) time.Sleep(1
* time.Second)
}
}func main()
{ go printNumbers() // Run printNumbers asynchronously
)
fmt.Println("Numbers are being printed in the background." time.Sleep(6 * time.Second) // Wait for the goroutine to finish
}
2. Communication Between Goroutines
Channels provide a way for goroutines to communicate with each other and synchronize their execution. By sending and receiving data through channels, you can ensure safe data sharing between goroutines.
package
mainimport
( "fmt"
)func sum(a int, b int, result chan int)
{
result <- a + b
}func main()
{ result := make(chan int
) go sum(1, 2
, result) fmt.Println("Sum:", <-result) // Receive the result from the channel
}
3. Distributing Work and Collecting Results
Using goroutines and channels, you can distribute tasks among multiple workers and collect their results. This pattern is especially useful for parallel processing.
package
mainimport
( "fmt"
)func worker(id int, jobs <-chan int, results chan<- int)
{ for j := range
jobs { fmt.Printf("Worker %d started job %d\n"
, id, j) results <- j * 2
, id, j)
fmt.Printf("Worker %d finished job %d\n"
}
}func main()
{ const numJobs = 5
, numJobs)
jobs := make(chan int results := make(chan int
, numJobs) for w := 1; w <= 3
; w++ { go
worker(w, jobs, results)
} for j := 1
; j <= numJobs; j++ {
jobs <- j
} close
(jobs) for a := 1
; a <= numJobs; a++ { fmt.Println("Result:"
, <-results)
}
}
4. Signaling Completion
Channels can also be used to signal the completion of a task. This is useful for synchronizing the end of goroutines with the main program.
package
mainimport
( "fmt"
"time"
)func worker(done chan bool)
{ fmt.Println("Working..."
) time.Sleep(2
* time.Second) fmt.Println("Done"
) done <- true
}func main()
{ done := make(chan bool, 1
) go
worker(done)
<-done
}
5. Multiplexing with Select Statement
The select
statement allows you to wait on multiple channel operations. This is useful for handling multiple concurrent events.
package
mainimport
( "fmt"
"time"
)func main()
{ ch1 := make(chan string
) ch2 := make(chan string
) go func()
{ time.Sleep(1
* time.Second) ch1 <- "one"
}() go func()
{ time.Sleep(2
* time.Second) ch2 <- "two"
}() for i := 0; i < 2
; i++ { select
{ case
msg1 := <-ch1: fmt.Println("Received"
, msg1) case
msg2 := <-ch2: fmt.Println("Received"
, msg2)
}
}
}
Conclusion
Goroutines and channels are powerful tools in Go for managing concurrency and communication between tasks. By understanding and utilizing these features, you can write efficient and scalable programs. Whether you're running asynchronous tasks, distributing workloads, or synchronizing operations, Go's concurrency model offers a robust solution to your programming needs.