Database Design for Social Login Functionality
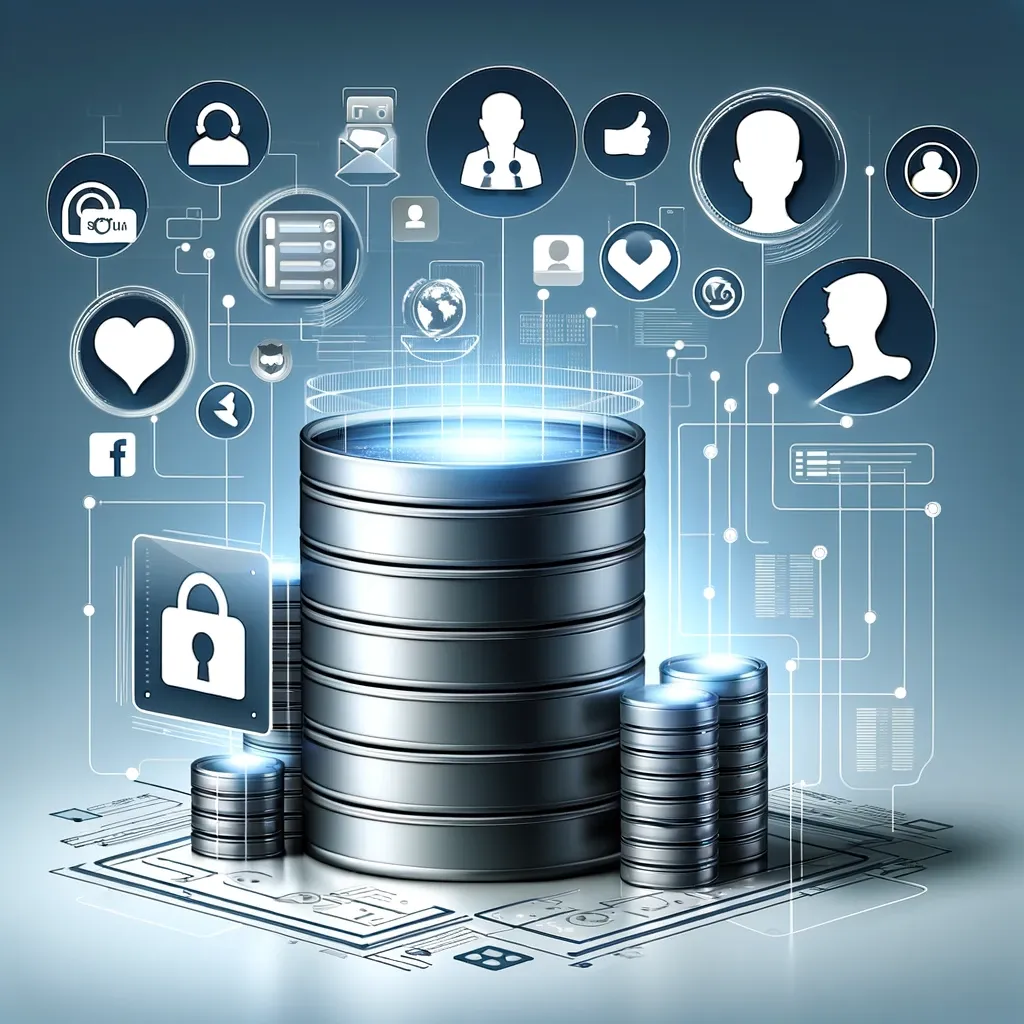
Introduction
Social login allows users to log into a website using their social media accounts like Facebook, Google, or Kakao. It simplifies the login process for users by eliminating the need for a separate registration process.
Table of Contents
- Introduction to Social Login
- Overview of Database Design
- User Table Design
- Social Account Table Design
- Example Code and SQL Queries
- Conclusion
1. Introduction to Social Login
Social login is a feature that lets users authenticate using their existing social media accounts. It enhances user convenience and can increase user registrations on your platform.
2. Overview of Database Design
To implement social login, we need a database design that can store both basic user information and social account details. Typically, this involves two tables:
- User Table
- Social Account Table
3. User Table Design
The User table stores basic information about users. Here's an example of how you might design this table:
CREATE TABLE User
( id INT PRIMARY
KEY AUTO_INCREMENT, username VARCHAR(50) NOT NULL
, email VARCHAR(100) NOT NULL UNIQUE
, password VARCHAR(255), -- Password may be null for social login users
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
4. Social Account Table Design
The Social Account table stores information about users' social media accounts. Here's an example design:
CREATE TABLE
SocialAccount ( id INT PRIMARY
KEY AUTO_INCREMENT, user_id INT NOT NULL
, provider VARCHAR(50) NOT NULL, -- Social login provider (e.g., 'facebook', 'google')
(id)
provider_id VARCHAR(100) NOT NULL, -- Unique ID from the social provider
FOREIGN KEY (user_id) REFERENCES User
);
This table links to the User table via user_id
and stores the provider name and unique ID from the social login provider.
ERD Diagram
Below is the Entity-Relationship Diagram (ERD) for the social login database design:
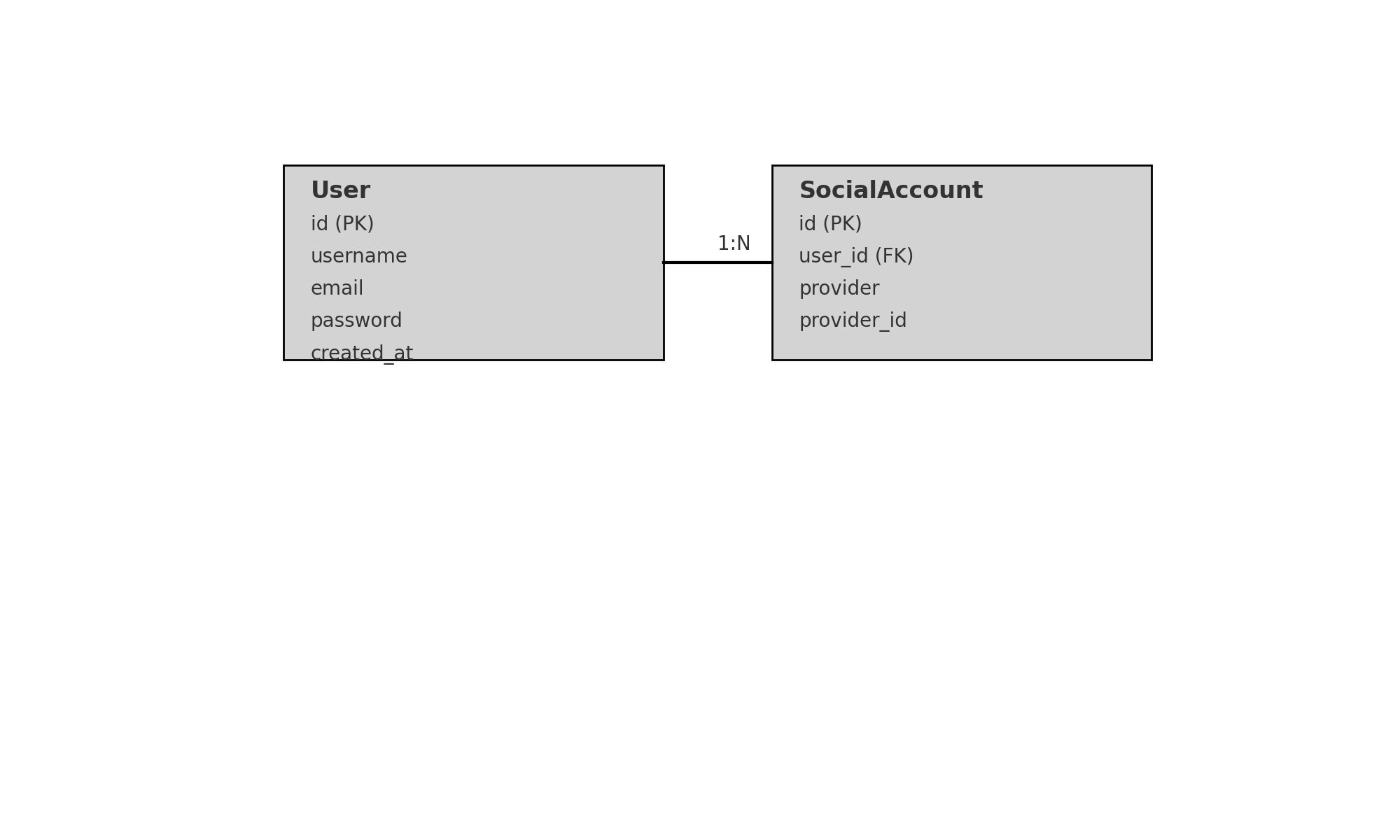
5. Example Code and SQL Queries
Here are some example SQL queries to add users and link social accounts:
- Adding a new user:
INSERT INTO User (username, email, password) VALUES ('exampleuser', 'user@example.com', 'password123'
);
- Linking a social account:
INSERT INTO SocialAccount (user_id, provider, provider_id) VALUES (1, 'google', '123456789'
);
- Retrieving user information along with their social accounts:
SELECT
u.username, u.email, s.provider, s.provider_idFROM User
uJOIN SocialAccount s ON u.id =
s.user_idWHERE u.id = 1
;
6. Conclusion
In this blog post, we covered the database design for implementing social login functionality. We designed the User and SocialAccount tables and provided example SQL queries for adding and retrieving data. This design should help you implement a robust social login system on your platform.