Building a Todo List App Using Golang and React
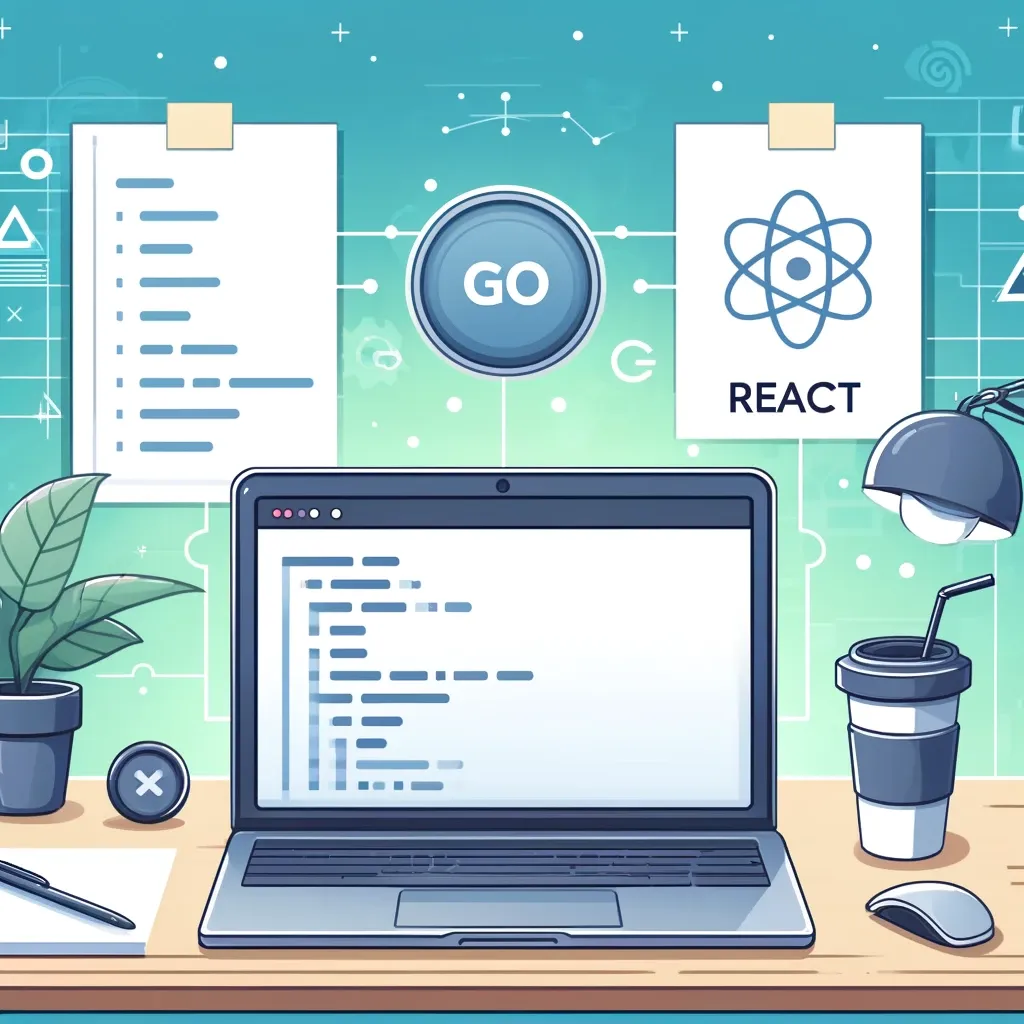
Creating a full-stack Todo List app is a great project to enhance your web development skills. In this blog post, I'll guide you through the process of building a Todo List application using Golang for the backend and React for the frontend. We'll cover everything from planning and development to deployment.
Project Planning
Objective
The goal is to create a simple yet functional Todo List application where users can add, delete, and view their tasks.
Tech Stack
- Backend: Golang (Gin framework)
- Frontend: React
- Database: MySQL
- Deployment: Docker
Backend Development with Golang
Setting Up the Environment
First, install Golang and set up the Gin framework. Create a new project directory and initialize it with Go modules.
mkdir
todo-appcd
todo-app
go mod init todo-app
go get -u github.com/gin-gonic/gin
Creating the Server
Set up the main server file (main.go
):
package
mainimport
( "github.com/gin-gonic/gin"
)func main()
{
router := gin.Default() router.GET("/todos"
, getTodos) router.POST("/todos"
, addTodo) router.DELETE("/todos/:id"
, deleteTodo) router.Run(":8080"
)
}
Implementing Handlers
Create handler functions for getting, adding, and deleting todos.
type Todo struct
{ ID int `json:"id"`
Title string `json:"title"`
}var
todos = []Todo{ {ID: 1, Title: "Learn Golang"
}, {ID: 2, Title: "Build a Todo App"
},
}func getTodos(c *gin.Context)
{ c.JSON(200
, todos)
}func addTodo(c *gin.Context)
{ var
newTodo Todo if err := c.ShouldBindJSON(&newTodo); err != nil
{ c.JSON(400, gin.H{"error"
: err.Error()}) return
} todos = append
(todos, newTodo) c.JSON(200
, newTodo)
}func deleteTodo(c *gin.Context)
{ id := c.Param("id"
) // Logic to delete a todo by ID
})
c.JSON(200, gin.H{"message": "Todo deleted"
}
Connecting to MySQL
Integrate MySQL to persist data.
import
( "database/sql"
_ "github.com/go-sql-driver/mysql"
)func setupDatabase() (*sql.DB, error
) { db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/todoapp"
) if err != nil
{ return nil
, err
} return db, nil
}
Frontend Development with React
Setting Up React
Use Create React App to set up the frontend.
npx create-react-app todo-frontendcd
todo-frontend
npm start
Creating Components
Build components for displaying and managing todos.
// TodoList.js
;
import React, { useState, useEffect } from 'react'function TodoList(
) { const [todos, setTodos] = useState
([]); useEffect(() =>
{ fetch('/todos'
) .then(response => response.json
()) .then(data => setTodos
(data));
}, []); return
( <div>
<h1>Todo List</h1>
<ul>
{todos.map(todo => ( <li key={todo.id}>{todo.title}</li>
))} </ul>
</div>
);
}export default TodoList
;
Adding New Todos
Create a form to add new todos.
// AddTodo.js
;
import React, { useState } from 'react'function AddTodo(
) { const [title, setTitle] = useState(''
); const handleSubmit = (e
) => { e.preventDefault
(); fetch('/todos'
, { method: 'POST'
, headers
: { 'Content-Type': 'application/json'
,
}, body: JSON.stringify
({ title }),
}) .then(response => response.json
()) .then(data =>
{ // Handle new todo
});
}; return
( <form onSubmit={handleSubmit}>
setTitle(e.target.value)}
<input
type="text"
value={title}
onChange={(e) =>
placeholder="New Todo"
/> <button type="submit">Add Todo</button>
</form>
);
}export default AddTodo
;
Deployment
Using Docker
Create Dockerfiles for the backend and frontend.
Backend Dockerfile
# Dockerfile for Golang backend
FROM golang:1.18-alpine
WORKDIR /app
COPY . .
RUN go build -o main .
CMD ["./main"]
Frontend Dockerfile
# Dockerfile for React frontend
FROM node:16-alpine
WORKDIR /app
COPY . .
RUN npm install
RUN npm run build
EXPOSE 3000
CMD ["npm", "start"]
Docker Compose
Create a docker-compose.yml
to manage both services.
version: '3'
services:
backend:
build: ./backend
ports:
- "8080:8080"
frontend:
build: ./frontend
ports:
- "3000:3000"
Conclusion
By following this guide, you will have a functional Todo List application using Golang and React. This project covers planning, development, and deployment, giving you a comprehensive overview of building a full-stack application. Happy coding!