Building a Telegram Bot with Go (Golang)
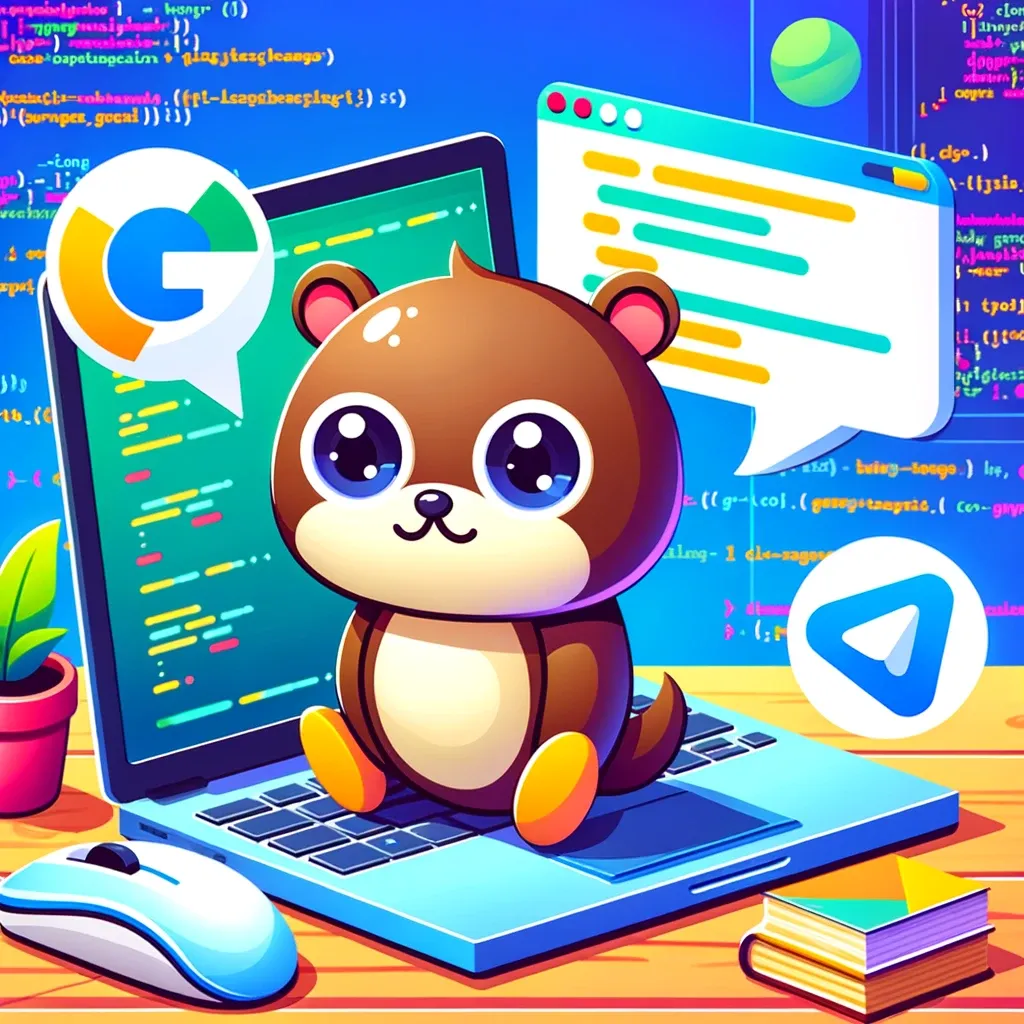
Hello, Go developers! In this post, we will explore how to build a Telegram bot using Go (Golang). Telegram bots are a great way to automate tasks, provide information, or even entertain users. Let's dive into the steps required to set up and develop a simple Telegram bot.
Introduction
Telegram bots can perform various tasks and interact with users through messages, commands, and inline requests. In this guide, we will create a simple bot that responds to user messages using the telebot
package in Go.
Prerequisites
- A Telegram account
- Go installed on your machine (version 1.12 or higher)
- Basic understanding of Go programming
Step 1: Create a New Bot on Telegram
- Open Telegram and search for the "BotFather" bot.
- Start a chat with BotFather and use the command
/newbot
. - Follow the prompts to set up your bot:
- Choose a name for your bot.
- Choose a username (it must end with
bot
, e.g.,my_awesome_bot
).
- Once the bot is created, BotFather will provide you with a token. Save this token securely as you will need it to access the Telegram API.
Step 2: Set Up Your Go Environment
- Create a new directory for your project:
mkdir
telegram-botcd
telegram-bot
- Initialize a new Go module:
go mod init telegram-bot
- Install the
telebot
package:
go get github.com/tucnak/telebot
Step 3: Write the Bot Code
Create a file named main.go
in your project directory and open it in your preferred code editor.
main.go
package
mainimport
( "log"
"time"
"github.com/tucnak/telebot"
)func main()
{ // Replace with your bot's token
botToken := "YOUR_TELEGRAM_BOT_TOKEN"
// Create a new bot instance
bot, err := telebot.NewBot(telebot.Settings{
Token: botToken, Poller: &telebot.LongPoller{Timeout: 10
* time.Second},
}) if err != nil
{
log.Fatal(err) return
} // Define the behavior for the /start command
{
bot.Handle("/start", func(m *telebot.Message) bot.Send(m.Sender, "Welcome! I am your Go Telegram bot."
)
}) // Define the behavior for text messages
{
bot.Handle(telebot.OnText, func(m *telebot.Message) bot.Send(m.Sender, "You said: "
+m.Text)
}) // Start the bot
)
log.Println("Starting bot..."
bot.Start()
}
Replace YOUR_TELEGRAM_BOT_TOKEN
with the token you received from BotFather.
Explanation
- We import necessary packages, including
telebot
for Telegram bot functionality. - We create a new bot instance using the provided token and set up a long poller to listen for updates.
- We define handlers for the
/start
command and text messages. - We start the bot, which begins polling for updates.
Step 4: Run Your Bot
- In your project directory, run the following command to start your bot:
go run main.go
- Open Telegram and start a chat with your bot by searching for its username.
- Send the
/start
command to see the welcome message. - Send any text message to see the bot echo back what you said.
Conclusion
Congratulations! You've built a simple Telegram bot using Go. This bot responds to the /start
command and echoes back any text messages. From here, you can extend the bot's functionality by adding more commands, integrating with APIs, and handling different types of messages.
If you have any questions or need further assistance, feel free to leave a comment. Happy coding!