Getting Started with go-redis in Go
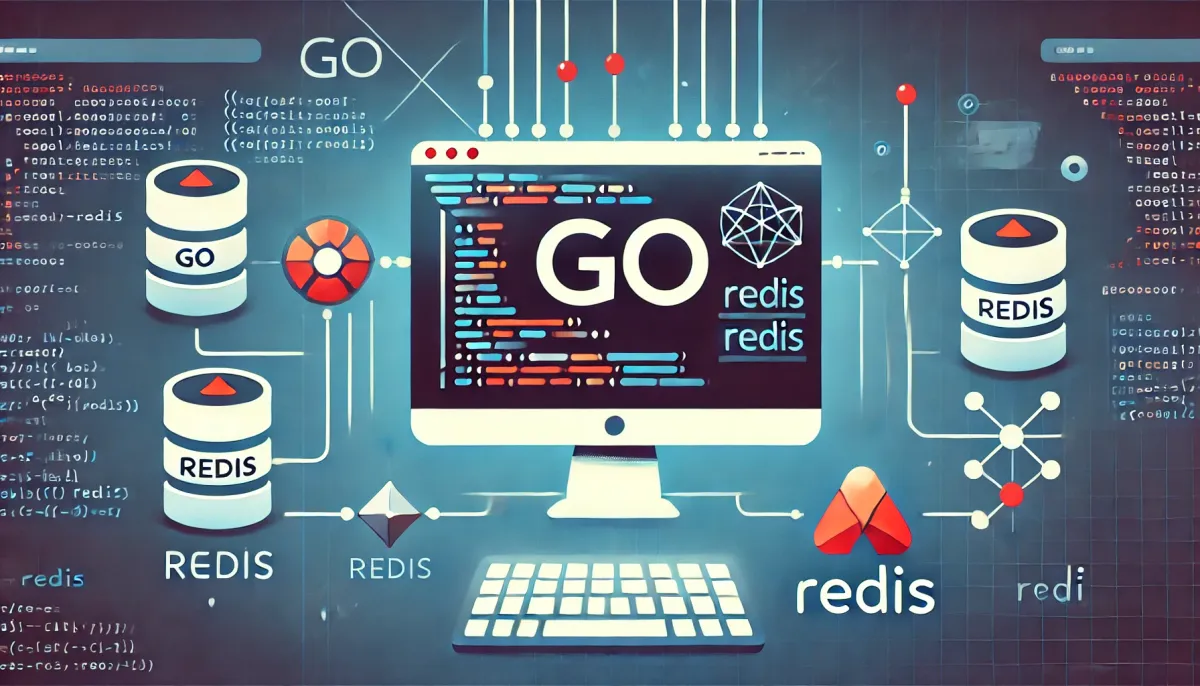
The go-redis
library is a powerful and easy-to-use client for working with Redis in Go. In this blog post, we will walk through the basics of setting up go-redis
, connecting to a Redis server, and executing some common Redis commands.
Installing go-redis
To get started, you need to install the go-redis
library. You can do this using go get
:
go get github.com/go-redis/redis/v8
Make sure to import the package in your Go file:
import
( "context"
"github.com/go-redis/redis/v8"
)
Connecting to Redis
The first step is to create a new Redis client and connect to your Redis server. Here is a simple example:
package
mainimport
( "context"
"fmt"
"github.com/go-redis/redis/v8"
)var
ctx = context.Background()func main()
{
rdb := redis.NewClient(&redis.Options{ Addr: "localhost:6379"
, Password: "", // no password set
DB: 0, // use default DB
})
pong, err := rdb.Ping(ctx).Result() if err != nil
{ fmt.Println("Could not connect to Redis:"
, err) return
} fmt.Println("Connected to Redis:"
, pong)
}
In this example, we create a new client connected to localhost
on port 6379
, with no password and the default database.
Basic Commands
SET and GET
The SET
command is used to set the value of a key, and the GET
command is used to retrieve the value of a key. Here is an example:
func main()
{
rdb := redis.NewClient(&redis.Options{ Addr: "localhost:6379"
, Password: ""
, DB: 0
,
}) err := rdb.Set(ctx, "key", "value", 0
).Err() if err != nil
{ fmt.Println("Error setting key:"
, err) return
} val, err := rdb.Get(ctx, "key"
).Result() if err != nil
{ fmt.Println("Error getting key:"
, err) return
} fmt.Println("key:"
, val)
}
INCR and DECR
The INCR
command increments the integer value of a key by one, and the DECR
command decrements the integer value of a key by one. Here is an example:
func main()
{
rdb := redis.NewClient(&redis.Options{ Addr: "localhost:6379"
, Password: ""
, DB: 0
,
}) err := rdb.Set(ctx, "counter", 0, 0
).Err() if err != nil
{ fmt.Println("Error setting counter:"
, err) return
} err = rdb.Incr(ctx, "counter"
).Err() if err != nil
{ fmt.Println("Error incrementing counter:"
, err) return
} counter, err := rdb.Get(ctx, "counter"
).Result() if err != nil
{ fmt.Println("Error getting counter:"
, err) return
} fmt.Println("counter:"
, counter)
}
List Operations: LPUSH and LRANGE
Redis lists are collections of strings sorted by insertion order. LPUSH
adds one or more values to the beginning of a list, and LRANGE
returns a range of elements from a list. Here is an example:
func main()
{
rdb := redis.NewClient(&redis.Options{ Addr: "localhost:6379"
, Password: ""
, DB: 0
,
}) err := rdb.LPush(ctx, "mylist", "world"
).Err() if err != nil
{ fmt.Println("Error pushing to list:"
, err) return
} err = rdb.LPush(ctx, "mylist", "hello"
).Err() if err != nil
{ fmt.Println("Error pushing to list:"
, err) return
} vals, err := rdb.LRange(ctx, "mylist", 0, -1
).Result() if err != nil
{ fmt.Println("Error getting list values:"
, err) return
} fmt.Println("mylist:"
, vals)
}
Hash Operations: HSET and HGET
Redis hashes are maps between string fields and string values. HSET
sets the value of a hash field, and HGET
gets the value of a hash field. Here is an example:
func main()
{
rdb := redis.NewClient(&redis.Options{ Addr: "localhost:6379"
, Password: ""
, DB: 0
,
}) err := rdb.HSet(ctx, "myhash", "field1", "value1"
).Err() if err != nil
{ fmt.Println("Error setting hash field:"
, err) return
} val, err := rdb.HGet(ctx, "myhash", "field1"
).Result() if err != nil
{ fmt.Println("Error getting hash field:"
, err) return
} fmt.Println("myhash field1:"
, val)
}
Conclusion
The go-redis
library provides a rich set of features for interacting with Redis. In this blog post, we've covered some basic commands to get you started. For more advanced usage and features, be sure to check out the official documentation.
By using these examples, you can quickly integrate Redis into your Go applications and start leveraging the power of Redis for your data storage needs.