Automating Go Project Tasks with Makefile
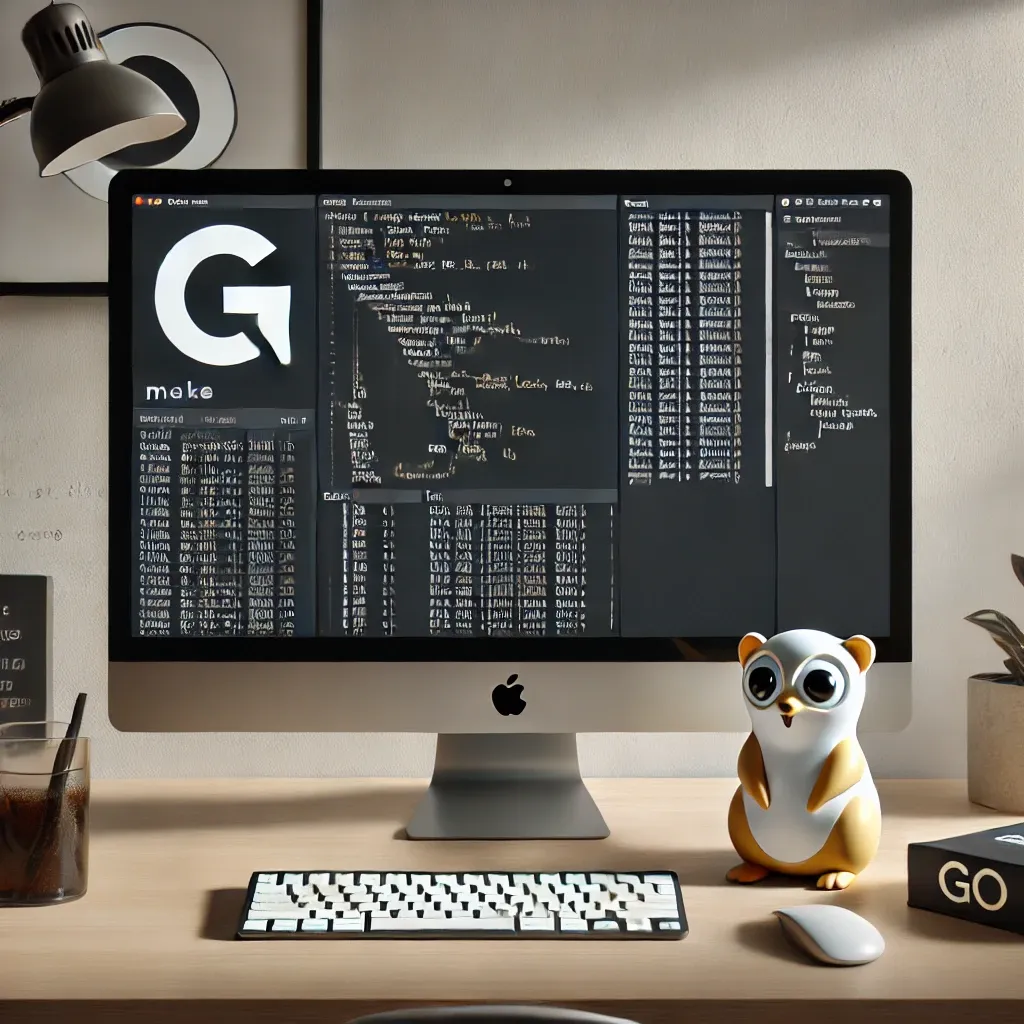
When working with a Go project, especially in a team or on larger projects, automating repetitive tasks such as building, testing, and cleaning up can save a lot of time. One of the simplest and most effective ways to achieve this is by using a Makefile
. While Makefiles are traditionally associated with C/C++ projects, they can be a powerful tool for managing Go projects as well.
In this post, we'll go through how to create a Makefile
for your Go project to streamline your development workflow.
What is a Makefile?
A Makefile
is a simple text file used to automate commands that you frequently run in your project. With a Makefile
, you can define sets of tasks (also called "targets") and run them using the make
command. This reduces the chance of mistakes and ensures consistency across different environments.
Creating a Basic Makefile for a Go Project
Let's start by writing a basic Makefile
to automate common tasks like building, testing, and cleaning up your Go project.
Step 1: Define Variables
In the Makefile
, you can define variables to manage paths, filenames, and other settings.
# Go project settings
APP_NAME := myapp
BUILD_DIR := ./bin
SRC_DIR := ./src
VERSION := 1.0.0
Here, APP_NAME
is the name of the output binary, BUILD_DIR
is the directory where the binary will be placed, and SRC_DIR
points to the directory where the source code resides.
Step 2: Define the Targets
Next, we define the tasks or "targets" that we want to automate. Below is a list of common tasks you might want to include in your Makefile
:
build
: Compile the project usinggo build
.test
: Run unit tests usinggo test
.clean
: Remove the binary and clean up the build folder.run
: Build and run the project.
Here is what the Makefile
would look like:
# Default target
all: build
# Build the Go application
build:
go build -o $(BUILD_DIR)/$(APP_NAME) $(SRC_DIR)
/main.go# Run tests
test:
go test ./...# Clean up binaries
clean:
rm -rf $(BUILD_DIR)
# Run the application
run: build
$(BUILD_DIR)/$(APP_NAME)
Step 3: Using the Makefile
Once your Makefile
is ready, you can use the make
command to execute any of the defined tasks:
- To build the application: make build
- To run the application: make run
- To test the application:
make test
- To clean the build folder: make clean
Step 4: Adding Go Module Management
If your project uses Go modules, you might want to add a task for managing Go dependencies. You can add a target that runs go mod tidy
:
# Tidy up Go modules
mod:
go mod tidy
Now, you can ensure that your project’s dependencies are clean by running:
make mod
Conclusion
A Makefile
is a great way to manage repetitive tasks in your Go project. By automating tasks like building, testing, and cleaning, you save time and avoid errors. Even though Go has its own powerful tools, using Makefile
gives you extra flexibility and control, especially when working in a team environment.
By setting up a Makefile
for your project, you ensure that tasks are consistently run across environments, making your development process more efficient.